The HTML Section
%%html
<style>
.Header{
background-color: aqua;
color: black;
font-family: cursive;
}
.p1{
background-color: darkorchid;
font-family:'Franklin Gothic Medium';
}
.p2{
background-color:darkred;
color:lightskyblue;
font-family: Impact;
}
</style>
<div>
<h3 class="Header">Web Programming HTML Test</h3>
<p class="p1">Want to get an annoying alert? Look no further!</p>
<button id="button" title="You sure?">Click me to get an alert!</button>
<script>
var button = document.getElementById("button");
button.addEventListener('click', function() {
alert("You clicked the button!");
});
</script>
</div>
<div>
<a href="https://media.discordapp.net/attachments/1143438030749847604/1156139470861647913/Man.png?ex=6513e249&is=651290c9&hm=0465268409fce7b051eeddea83e6a1efb9ac5441e4babe5cb048c99714603ef7&=&width=973&height=605" target="_blank" title="Thank you for">Random meme 1</a>
<br>
<a href="https://media.discordapp.net/attachments/1143438030749847604/1156140085243293797/Intimidation_is_key.jpg?ex=6513e2dc&is=6512915c&hm=ede45e69c7d5c19595f12e5437867a1b4192869a26f5a0e8837fddcf17bf6f16&=&width=636&height=605" target="_blank" title="reading the below text!">Random meme 2</a>
<p class="p2">Above you will see two links. Hover over them for a special message, and click them to go to random memes I found in my computer at 1 am.</p>
</div>
<div>
<p>Also, potential project idea????</p>
<img alt="Dark Souls" src="https://cdn.cloudflare.steamstatic.com/steam/apps/374320/capsule_616x353.jpg?t=1682651227" width="300" height="200">
<p>but like terminal and not very aesthetic version</p>
</div>
Web Programming HTML Test
Want to get an annoying alert? Look no further!
Random meme 1
Random meme 2
Random meme 2
Above you will see two links. Hover over them for a special message, and click them to go to random memes I found in my computer at 1 am.
Also, potential project idea????
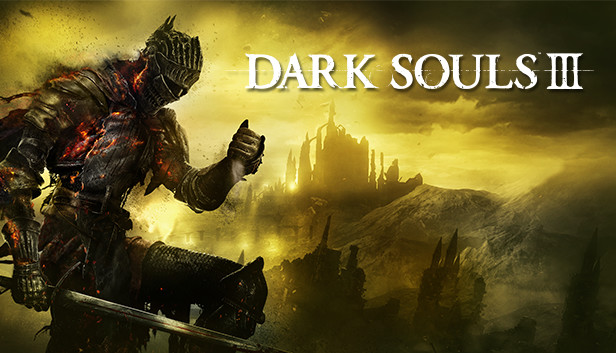
but like terminal and not very aesthetic version
The Data Types Section
%%js
// Define object
var currentclassesvalue = ["AP Statistics", "AP CSP", "AP Biology", "APUSH", "APEL"]
var interestsvalue = ["Chemistry and science in general", "Trumpet and classical music", "Anime and manga", "Games", "Reading"]
var ageofteamvalue = [16, 14, 17, 17]
var me = {
name: "Jason Gao",
age: 16,
birthdate: "Decembler 22nd, 2006",
currentclasses: currentclassesvalue,
interests: interestsvalue,
ageofteam: ageofteamvalue
}
// Output to console
console.log("Here is an object that represents me.")
console.log("It includes my name, age, birthday, the current classes I'm taking, a few of my interestings, and the age of my team members in the Team Test.")
console.log(me)
// Changes to make
console.log("However, I forgot to add my own age to the array with the ages of our team in the Team Test. I also would like to swap an interest to a new one in my array of interests. Lastly, I made a typo in writing my birthday, so I would like to fix that.")
// Add my age to ageofteam
console.log("Adding age...")
ageofteamvalue.push(16)
// Swap interest
console.log("Swapping interest...")
interestsvalue.pop()
interestsvalue.push("History")
// Fix typo
console.log("Fixing typo...")
me.birthdate = "December 22nd, 2006"
// Print fixed objects
console.log("All the edits have been made, here is the fixed object:")
console.log(me)
// Average age of team
console.log("The object is now fixed, so we can now examine parts of this object.")
console.log("I work in a team of 5, and below is the math for the average age of our team.")
var sumofage = ageofteamvalue[0] + ageofteamvalue[1] + ageofteamvalue[2] + ageofteamvalue[3] + ageofteamvalue[4]
console.log("The sum of everyone's ages is " + sumofage.toString())
var avgage = sumofage / 5
console.log("The average of everyone's ages is " + avgage.toString())
// Data types
console.log("The datatype of the variable name is " + typeof me.name)
console.log("The datatype of the variable age is " + typeof me.age)
console.log("The datatype of the variable birthdate is " + typeof me.birthdate)
console.log("The datatype of the varaible currentclasses is " + typeof currentclassesvalue)
console.log("The datatype of the variable interests is " + typeof interestsvalue)
console.log("The datatype of the variable ageofteam is " + typeof ageofteamvalue)
The DOM Section (Must show in VSCode)
%%html
<style>
.Header{
background-color: aqua;
color: black;
font-family: cursive;
}
.p1{
background-color: darkorchid;
font-family:'Franklin Gothic Medium';
}
.p2{
background-color:darkred;
color:lightskyblue;
font-family: Impact;
}
</style>
<div>
<h3 class="Header">DOM Section</h3>
<p class="p1" id="paragraph1">Click the button below to swap the links the below!</p>
<button id="button" title="Click to swap!">Click me to swap the links!</button>
<script>
var button = document.getElementById("button");
button.addEventListener('click', function() {
var paragraph1element = document.getElementById("paragraph1");
paragraph1element.innerHTML = "Switched!";
var link1 = document.querySelector("a[href='https://media.discordapp.net/attachments/1143438030749847604/1156139470861647913/Man.png?ex=6513e249&is=651290c9&hm=0465268409fce7b051eeddea83e6a1efb9ac5441e4babe5cb048c99714603ef7&=&width=973&height=605']");
var link2 = document.querySelector("a[href='https://media.discordapp.net/attachments/1143438030749847604/1156140085243293797/Intimidation_is_key.jpg?ex=6513e2dc&is=6512915c&hm=ede45e69c7d5c19595f12e5437867a1b4192869a26f5a0e8837fddcf17bf6f16&=&width=636&height=605']");
var copylink1 = link1.href;
link1.href = link2.href;
link2.href = copylink1;
var copytext1 = link1.textContent;
link1.textContent = link2.textContent;
link2.textContent = copytext1;
});
</script>
</div>
<div>
<a href="https://media.discordapp.net/attachments/1143438030749847604/1156139470861647913/Man.png?ex=6513e249&is=651290c9&hm=0465268409fce7b051eeddea83e6a1efb9ac5441e4babe5cb048c99714603ef7&=&width=973&height=605" target="_blank" title="Thank you for">Random meme 1</a>
<br>
<a href="https://media.discordapp.net/attachments/1143438030749847604/1156140085243293797/Intimidation_is_key.jpg?ex=6513e2dc&is=6512915c&hm=ede45e69c7d5c19595f12e5437867a1b4192869a26f5a0e8837fddcf17bf6f16&=&width=636&height=605" target="_blank" title="reading the below text!">Random meme 2</a>
<p class="p2">Above you will see two links. Click the button to swap the two links! Note that these are links to random memes I found in my computer at 1 am.</p>
</div>
DOM Section
Click the button below to swap the links the below!
Random meme 1
Random meme 2
Random meme 2
Above you will see two links. Click the button to swap the two links! Note that these are links to random memes I found in my computer at 1 am.
The JavaScript Section
%%html
<style>
.DarkSouls{
background-color: snow;
color: crimson;
font-size: large;
font-family: fantasy;
}
</style>
<h3 class="DarkSouls">Game Health Calculator</h3>
<p class="DarkSouls">DUN DUN DUNNN. DARK SOULS BOSS. YOU HAVE 10 HP. THE BOSS HITS YOU.</p>
<p>Please see the console for everything, the above text has no function :D</p>
<script>
var a = 10
var b = 15
console.log("Let's say you start with 10 HP, so this is variable a")
console.log("Let's say the boss hits you for 15 damage, so this is variable b")
if (a > b) {
console.log("a is greater than b. Therefore, your health is greater than the damage taken, so you live in the game.")
}
if (a == b) {
console.log("both a and b are equal. Therefore, you took the same amount of damage as your health and unfortunately died in the game.")
}
if (a < b) {
console.log("b is greater than a. Therefore, you took more damage than your health and unfortunately died in the game.")
}
console.log("Mess around with the variable values in VSCode and see the different outputs!")
</script>
The JS Debugging Section
Segment 1: Alphabet List
See code below
%%js
// ORIGINAL CODE
// var alphabet = "abcdefghijklmnopqrstuvwxyz";
// var alphabetList = [];
// for (var i = 0; i < 10; i++) {
// alphabetList.push(i);
// }
// console.log(alphabetList);
// FIXED CODE
var alphabet = "abcdefghijklmnopqrstuvwxyz";
var alphabetList = [];
for (var i = 0; i < alphabet.length; i++) {
alphabetList.push(alphabet[i]);
}
console.log(alphabetList);
What I changed:
- Instead of limiting the upper bound of i to less than 10, I limited it to the end of the length of the alphabet string so the code would actually iterate through the entirety of the alphabet string by turning “i < 10” into “i < alphabet.length”
- Rather than pushing i, which is the number, I pushed the character in the alphabet string corresponding to the value of i by turning “alphabetList.push(i)” into “alphabetList.push(alphabet[i])”
Segment 2: Numbered Alphabet
See code below
%%js
// Copy your previous code to built alphabetList here
var alphabet = "abcdefghijklmnopqrstuvwxyz";
var alphabetList = [];
for (var i = 0; i < alphabet.length; i++) {
alphabetList.push(alphabet[i]);
}
// ORIGINAL CODE
// let letterNumber = 5
// for (var i = 0; i < alphabetList; i++) {
// if (i === letterNumber) {
// console.log(letterNumber + " is letter number 1 in the alphabet")
// }
// }
// Should output:
// "e" is letter number 5 in the alphabet
// FIXED CODE
let letterNumber = 5
for (var i = 0; i < alphabet.length; i++) {
if (i === letterNumber) {
var letter = alphabet[letterNumber-1];
console.log(letter + " is letter number " + letterNumber + " in the alphabet")
}
}
What I changed:
- First I changed the iteration to iterate from 0 to less than the length of the alphabet rather than the alphabet list, so it would actually go through each character in the alphabet string. I did this by changing “i < alphabetList” to “i < alphabet.length”
- Second, I set a new variable “letter” to be the actual letter in the alphabet string at the position of i, or letterNumber. I then outputted this new variable instead of letterNumber directly so it actually outputted the letter rather than the number. I did this by adding “var letter = alphabet[letterNumber-1]”. Note that the letterNumber is being subtracted by 1 because the code uses 0 based counting while our counting is 1 based counting, the letterNumber will be 1 ahead so I subtract by 1.
- Lastly, I edited the output text and used concatenation to add the letterNumber variable directly rather than a static number by changing 1 into letterNumber and doing the appropriate concatenation.
Segment 3: Odd Numbers
See code below
%%js
// ORIGINAL CODE
// let evens = [];
// let i = 0;
// while (i <= 10) {
// evens.push(i);
// i += 2;
// }
// console.log(evens);
// FIXED CODE
let odd = [];
let i = 1;
while (i <= 10) {
odd.push(i);
i += 2;
}
console.log(odd);
What I changed:
- The programs code was intended to print the odd numbers below 10, so first to make the purpose more clear, I changed the array name to odd
- Then in order to get all the odd numbers, I changed the “i = 0” to “i = 1”, so the first odd number would be correctly set to 1 rather than 0, so when “i += 2” happens, it adds 2 to 1 (to give 3, the next odd number) rather than adding 2 to 0 (to give 2, the wrong number).
Below Not Edited
See code below
- Most values are outputted incorrectly as numbers that are both divisible by 5 and 2 satisfy both if conditions, so the program pushes that number twice (one time from the if divisible by 5, and a second time from the if divisible by 2). Therefore, the output includes 10 twice, 20 twice, 30 twice, and so on.
- This change is thankfully very simple. The goal is to not have both conditions run if both are fulfilled. This can be accomplished by changing the second if condition to else if, so the code under the second else if condition won’t run if the first if condition is fulfilled.
%%js
var numbers = []
var newNumbers = []
var i = 0
while (i < 100) {
numbers.push(i)
i += 1
}
for (var i of numbers) {
if (numbers[i] % 5 === 0)
newNumbers.push(numbers[i])
else if (numbers[i] % 2 === 0)
newNumbers.push(numbers[i])
}
console.log(newNumbers)
Challenge
See code below
%%js
var menu = {"burger": 3.99,
"fries": 1.99,
"drink": 0.99}
var total = 0
// Shows menu and prompt for item
console.log("Menu")
for (var item in menu) {
console.log(item + " $" + menu[item].toFixed(2)) // toFixed(2) makes it so the number has 2 decimals
}
while (ask !== "no") {
var ask = prompt("What would you like from the menu? If you're finished, please enter no");
if (ask == "burger") {
total += menu["burger"]
}
}
// Total Price
console.log(total)
<IPython.core.display.Javascript object>